In this article we will cover how to populate or bind Dependent Dropdown with two way data binding in Blazor webassembly Application.
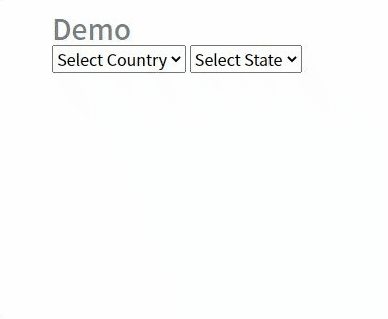
Models
Create a below mentioned class inside "Models" Folder
public class DemoModel { public List<Country> countries { get; set; } = new List<Country>(); public List<State> states { get; set; } = new List<State>(); public string Country_Code { get; set; } public string State_Code { get; set; } } public class Country { public string Country_Title { get; set; } public string Country_Code { get; set; } } public class State { public string State_Title { get; set; } public string State_Code { get; set; } public string Country_Code { get; set; } }
Demo.razor.cs
Create a class named "Demo.razor.cs" and add below mentioned code.
using Microsoft.AspNetCore.Components; using System; using System.Collections.Generic; using System.Linq; using System.Threading.Tasks; namespace HpBlogs.Pages { public partial class Demo : ComponentBase { public DemoModel model { get; set; } = new DemoModel(); protected async override Task OnInitializedAsync() { model.countries.Add(new Country() { Country_Code = "IN", Country_Title = "India" }); model.countries.Add(new Country() { Country_Code = "USA", Country_Title = "USA" }); model.countries.Add(new Country() { Country_Code = "FR", Country_Title = "France" }); model.countries.Add(new Country() { Country_Code = "BR", Country_Title = "Brazil" }); // POPULATE COUNTRY LIST FROM DATABASE USING API } protected async Task Country_Change(string cCode) { Console.WriteLine(cCode + "cCode"); model.Country_Code = cCode; model.State_Code = ""; List<State> statelist = new List<State>(); statelist.Add(new State() { State_Code = "GJ", State_Title = "Gujarat", Country_Code = "IN" }); statelist.Add(new State() { State_Code = "MP", State_Title = "Madhya Pradesh", Country_Code = "IN" }); statelist.Add(new State() { State_Code = "NR", State_Title = "New York", Country_Code = "USA" }); statelist.Add(new State() { State_Code = "BR", State_Title = "Berry", Country_Code = "FR" }); statelist.Add(new State() { State_Code = "BH", State_Title = "Bahia", Country_Code = "BR" }); // POPULATE STATE LIST FROM DATABASE USING API model.states = statelist.Where(w => w.Country_Code.Equals(cCode)).ToList(); } } }
Demo.razor
Create a page named "Demo.razor" and add below mentioned html
@page "/demo" <div class="row"> <EditForm Model="model"> <h3>Demo</h3> <InputSelect ValueExpression="@(()=>model.Country_Code)" Value="@model.Country_Code" ValueChanged="@((string cCode) => Country_Change(cCode))" placeholder="Country"> <option value="">Select Country</option> @if (model.countries != null) { @foreach (var item in model.countries) { <option value="@item.Country_Code">@item.Country_Title</option> } } </InputSelect> <InputSelect @bind-Value="model.State_Code" placeholder="State"> <option value="">Select State</option> @if (model.states != null) { @foreach (var item in model.states) { <option value="@item.State_Code">@item.State_Title</option> } } </InputSelect> </EditForm> </div>
Post Comments(0)