In this article we will cover how to pass model data to partial component or page with two way data binding in Blazor webassembly Application.
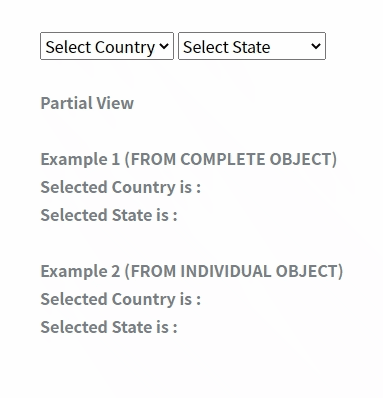
Models
Create a below mentioned class inside "Models" Folder
public class DemoModel { public List<Country> countries { get; set; } = new List<Country>(); public List<State> states { get; set; } = new List<State>(); public string Country_Code { get; set; } public string State_Code { get; set; } } public class Country { public string Country_Title { get; set; } public string Country_Code { get; set; } } public class State { public string State_Title { get; set; } public string State_Code { get; set; } }
Demo.razor.cs
Create a class named "Demo.razor.cs" and add below mentioned code.
using HpBlogs.Models; using Microsoft.AspNetCore.Components; using System.Collections.Generic; using System.Threading.Tasks; namespace HpBlogs.Pages { public partial class Demo : ComponentBase { public DemoModel model { get; set; } = new DemoModel(); protected async override Task OnInitializedAsync() { model.countries.Add(new Country() { Country_Code = "India", Country_Title = "India" }); model.countries.Add(new Country() { Country_Code = "USA", Country_Title = "USA" }); model.countries.Add(new Country() { Country_Code = "France", Country_Title = "France" }); model.countries.Add(new Country() { Country_Code = "Brazil", Country_Title = "Brazil" }); model.states.Add(new State() { State_Code = "Gujarat", State_Title = "Gujarat" }); model.states.Add(new State() { State_Code = "Madhya Pradesh", State_Title = "Madhya Pradesh" }); model.states.Add(new State() { State_Code = "New York", State_Title = "New York" }); model.states.Add(new State() { State_Code = "Berry", State_Title = "Berry" }); model.states.Add(new State() { State_Code = "Bahia", State_Title = "Bahia" }); } } }
Partial.razor
Create a page named "Partial.razor" and add below mentioned html
<strong>Partial View</strong> <strong>Example 1 (FROM COMPLETE OBJECT)</strong> <b>Selected Country is : @Data.Country_Code</b> <b>Selected State is : @Data.State_Code</b> <strong>Example 2 (FROM INDIVIDUAL OBJECT)</strong> <b>Selected Country is : @Country</b> <b>Selected State is : @State</b> @code{ [Parameter] public DemoModel Data { get; set; } = new DemoModel(); //COMPLETE OBJECT [Parameter] public string Country { get; set; } //INDIVIDUAL OBJECT [Parameter] public string State { get; set; } //INDIVIDUAL OBJECT protected override Task OnInitializedAsync() { return base.OnInitializedAsync(); } }
Demo.razor
Create a page named "Demo.razor" and add below mentioned html
@page "/demo" <section class="dashboard-area"> <div class="dashboard-content-wrap"> <div class="container-fluid"> <div class="row"> <EditForm Model="model"> <InputSelect @bind-Value="model.Country_Code" placeholder="Country"> <option value="">Select Country</option> @if (model.countries != null) { @foreach (var item in model.countries) { <option value="@item.Country_Code">@item.Country_Title</option> } } </InputSelect> <InputSelect @bind-Value="model.State_Code" placeholder="State"> <option value="">Select State</option> @if (model.states != null) { @foreach (var item in model.states) { <option value="@item.State_Code">@item.State_Title</option> } } </InputSelect> @*Partial Component / Page*@ <Partial Data="model" Country="@model.Country_Code" State="@model.State_Code" /> </EditForm> </div> </div> </div> </section>
Post Comments(0)